There is a way to produce sound that is simple and processing power efficient, this is using MIDI. This is a guide to use MIDI and generate sound with your Raspberry Pi. The resulting sound is not nearly as neat as a native synthesizer, but this will give you a glance of what you can do using Midi and your Raspberry Pi.
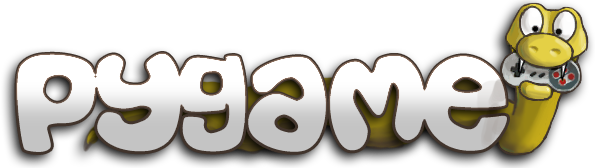
MIDI
So, if you are new to this subject I recommend you to look the MIDI Wiki. It´s a great way to create music, you´ll not regret it. This guide will not try to explain what the wiki page already did.
Basically, to create a MIDI note you´ll have to specify some parameters. This guide will only focus on the very basic parameters. You´ll need:
- MIDI note: The note that will be played.
- Velocity: How "loud" will the note play.
- Instrument: The kind of musical instrument to play.
When a note is going to be played, there is the need to create a note_on message. This will indicate that the note with the current saved parameters (note, velocity, instrument, etc.) will be heard. When is time to turn the note off, a note_off message is issued and the sound will stop. Note that some instruments (like piano, guitar, etc.) does not have a very long sustain, so even if a note_off is not issued the sound will fade. This does't mean that a note_off message should not be executed.
Software
There is a good chance that Pygame python module is already installed in your Raspbian image. You can look for it using a command in python shell/promt.
>>> help('modules')
This command will list all the python modules available in your python installation. If Pygame is not installed, I suggest that do a Google search. There are a great number of tutorials and guides on how to do this. Ask me anytime if you run into trouble.
Just run or download the following code.
# Derick DeLeon
# 2014-01
# midiHelloWorld.py
# This is a simple program the plays 128 notes using Pygames Midi module
import pygame
import pygame.midi
from time import sleep
# Parameters that you can change
instrument = 0
velocity = 127
# Initialize the Pygame and pygame.midi modules
pygame.init()
pygame.midi.init()
# This port number seems the only one to work
port = 2
latency = 1
# Set parameters
midiOutput = pygame.midi.Output(port, latency)
midiOutput.set_instrument(instrument)
# Play all 128 notes
for note in range(0, 127):
midiOutput.note_on(note, velocity)
sleep(.25)
midiOutput.note_off(note, velocity)
# close the handler and quit midi
del midiOutput
pygame.midi.quit()
Now change settings, play other notes and create your favorite song. For more information, please refer to General MIDI Standard. There you can find an instrument chart and the note number reference.
This comment has been removed by a blog administrator.
ReplyDeleteI get no audio out on either port 0 or port 2. Others have this problem: https://www.raspberrypi.org/forums/viewtopic.php?f=28&t=116715&p=856931
ReplyDeleteHelpfull post , thanks for shearing this .
ReplyDelete